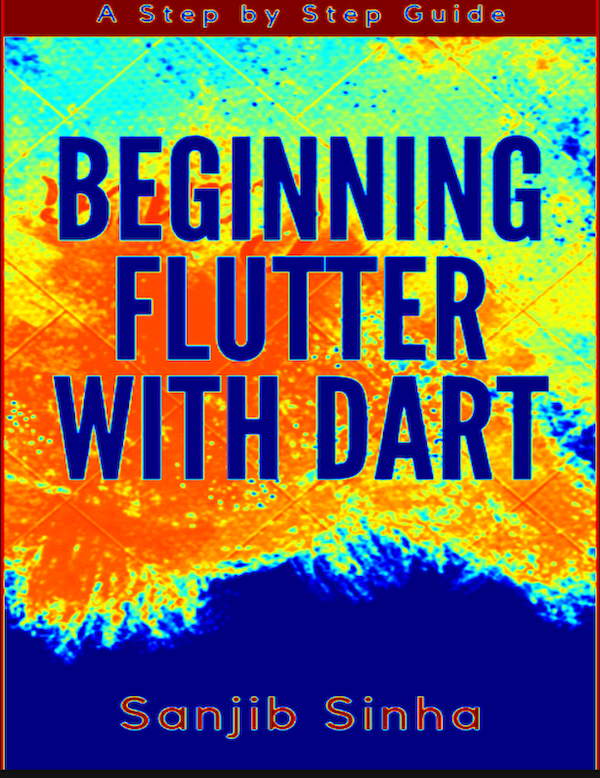
Who should read this Beginning Flutter with Dart Step by Step Guide book?
Are you an absolute beginner who without having any prior knowledge of programming language wants to build a mobile application? Well, then this book is for you. This book is not for intermediate or experienced learners or developers.
We will try to add two things to our knowledge so that we will start building your mobile application. First we will learn Flutter, a framework or tool that helps us to build the mobile application. Second, we will learn a programming language called Dart, with which Flutter works.
If we do not understand the basic syntax and semantics of Dart, we will not be able to understand the internal activities of Flutter. We will learn both, Flutter and Dart side by side. For instance, if we find something like function and object or named parameters in a constructor, we will learn that concept in Dart.
If you have no knowledge of programming language, or you have not written a single line of code, you need not worry. We will go very smooth, we will have plenty of screenshots that will explain what we are going to do. We will also learn the basic concepts of programming language through Dart; it is important, because otherwise we will not be able to understand how Flutter framework works.
DOWNLOAD Beginning Flutter with Dart Step by Step Guide PDF
DOWNLOAD Beginning Flutter with Dart Step by Step Guide EPUB
DOWNLOAD Beginning Flutter with Dart Step by Step Guide Mobile
Flutter and Dart Architecture: Understanding Class and Object
So far, we have understood one key concept. Flutter has many in-built classes and objects that interacting with each other and build application. This is a complex process and it runs on Dart platform.
We have also seen that in any Dart code, ‘package’ or libraries play the key role. In a Flutter project, everything runs through the ‘main.dart’ file, or through the main() function.
Now it is impossible to write hundreds of classes and creating thousands of objects inside one ‘main.dart’ file. The key concept of object-oriented programming is modularity. Breaking the application into several different parts will enhance the ability to organize our code. Therefore, we need to understand the key concepts of class and objects.
A Short Introduction to Class and Objects
As we know, Dart is an object-oriented language with classes and objects. Every object is an instance of a class and all classes descend from Object.
For absolute beginners, it is a conflicting statement. Class is behind every object. And it says, behind classes there are objects. What does it actually mean? It means a class could have many classes inside. It can inherit properties and methods of other classes, when that class has an instance or object, we can say that the object is behind many classes.
In Dart, there is a concept called ‘Mixin-based’ inheritance. It means every class has exactly one superclass, and a class body can be reused in multiple hierarchies. This concept is a little bit advanced for absolute beginners. So we will cover ‘Mixin-based’ inheritance at the end of this Beginning Flutter with Dart Step by Step Guide book.
To begin with, we start with a simple class and an object. So far we have seen variables and functions. We have seen how we can pass variables as parameters.
Let us think about something that will hold variables and functions inside. We call it a class. You should think this way: an object has states, like a person object has ‘colorOfEyes’. It is a state. A state can always be changed. Therefore, an object can also change its state. So, we can conclude that an object has some states and it can also change its states.
The way or method that are used in changing object-states are called ‘methods’. You can also call it ‘function’. Many languages use the keyword function, and some other use the term method. Extending our person object, which has state like ‘colorOfEyes’, we can say that the person object has one method called ‘wearContactLens()’. Now, using that method, the person object can change its state ‘colorOfEyes’
Flutter and Dart Architecture: Understanding Class and Object 25
A class always acts like a blueprint of an object. What will be the states, and how those states can be changed, all are predefined in a class.
In the next section we will see a long list of code, which will create mainly two objects; a person from a Person class, and a robot object from a Robot class. If you do not understand it at the first glance, do not worry. It takes time.
How two objects interact
Before starting to learn how two objects interact, we must remember that Dart is an object-oriented programming language. It means, in Dart, everything is an object.
Behind every object, there is a blueprint or class that decides how an object will change its states using different methods. We will discuss about objects in more detail, as we progress. For the sake of simplicity, you only know that we are all objects. Actually, any object-oriented language simulates the real world.
Here, in the coming application mobile game, we assume that one person object owns one robot object. The person object and the robot object have names, and they can do some actions. An object ideally should have a state and it can also change its state by using methods.
Consider a simple example. Besides having names, the robot object has state like ‘number of bullets’ that it can fire at something. We can change the state of the number of bullets by using a method called ‘canFire(number of bullets)’, passing the number of bullets as its parameter.
When a person owns a robot, he or she can use it to fire at some other person objects. And the person object that has been fired at, can strike back to the person object who fires at him.
By now, you understand that we are talking about four different objects. They are interacting with one another. In any application, be it a software, or a mobile application, or a web application, these interactions between various objects keep going on.
Our, programmer’s job is to design the application most efficiently. Okay, enough talking, let us watch some code now. Here is the first code snippet, where we have written two classes, Person and Robot. We have also created two objects, belonging to each of them. In the bottom section, we have commented out some actions that we are going to write in the next code snippet.